ExceptCXX, is a C++ exception library that is a superset of the C++ standard exception class std::exception. Let us take a look at what it supports and how to integrate it with your project.
The library has support for extracting the stack trace for when the throw was invoked, allowing for an easier development process whenever your code fails, without requiring to use the debugger of your liking, though using a debugger is still a recommendation. Since ExceptionCXX stack trace does not replace, just add more information when a program fails.
Furthermore, it can for instance be used for deployed software that clients are perhaps using and is an easy way to extract and pass over to the developer.
Integrate with current Standard library
The library was designed so that it would not be required to reimplement exception handling in your code, instead, it can simply be extended if needed. See the following example.
Here the code throws an exception and it is caught as a simple standard c++ exception. However, passing the exception object to the printStackMessage or getStackMessage, from the ExceptCXX library. It can extract more information such as stack trace, type of exception and etc.
try { throw cxxexcept::DivideByZeroException(); } catch (const std::exception &ex) { cxxexcept::printStackMessage(ex); }
A complete C++ executable program example.
int main(int argc, const char **argv) { try { throw cxxexcept::DivideByZeroException(); } catch (const std::exception &ex) { std::cerr << cxxexcept::getStackMessage(ex); return EXIT_FAILURE; } return EXIT_SUCCESS; }
Integrate with CMake
If using CMake as your build configuration tool, it can easily be integrated with little effort.
First, add the directory to the ExceptCXX Root directory to the ADD_SUBDIRECTORY, followed by the EXCLUDE_FROM_ALL. THe EXCLUDE_FROM_ALL will prevent make from running all the targets in the CMake file, like test and etc, which is mostly not of interest when integrating with your project.
Finally, for either your executable or library, ExceptCXX can be added by using the TARGET_LINK_LIBRARIES, which takes the library/executable as the first argument, followed by the cxxexcept.
ADD_SUBDIRECTORY(exceptCXX EXCLUDE_FROM_ALL) ... ... ... TARGET_LINK_LIBRARIES(myTarget PUBLIC cxxexcept)
The PUBLIC sets the scope, which is related to if myTarget for instance is a library and is linked to another target, if PUBLC, it will pass the cxxexcept, however, if PRIVATE, it will not pass cxxexcept, and it might fail.
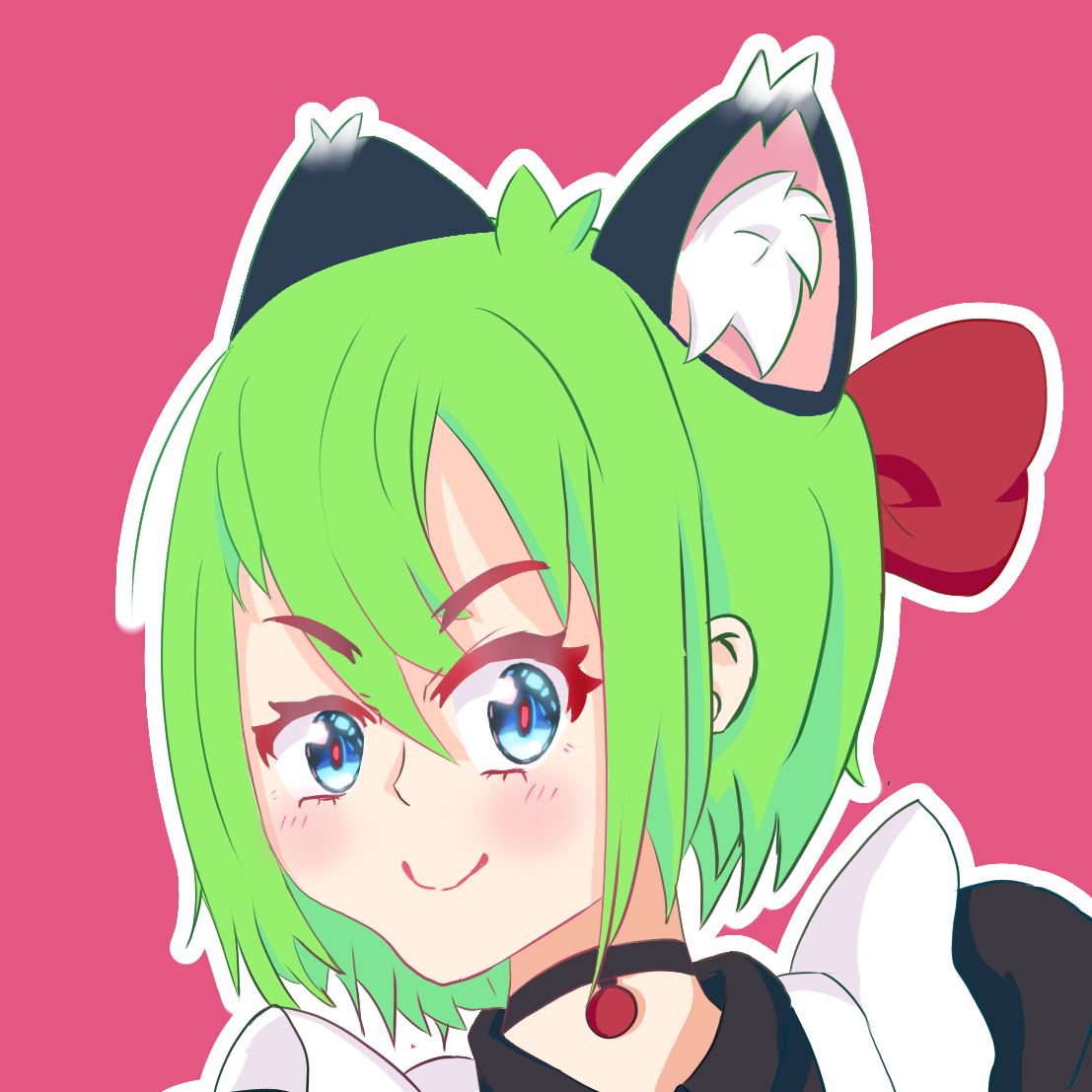
Free/Open software developer, Linux user, Graphic C/C++ software developer, network & hardware enthusiast.