When starting an android app, it is important to give a good impression by having a good looking splash screen during loading the app. There are multiple ways it can be created, this approach will use the dedicated activity and a drawable XML file. Where the drawable XML will be used as a theme style. The source code and the project can be found on GitHub. See the following example for how the splash screen can look like when starting an Android app.
Setup Splash Drawable
First, setup the splash drawable can be done with an XML file. The first item, adds the background color, the second item adds the bitmap image. The android:tileMode prevents the image from being tiled. Though, the most important attribute is the android:src. The source file has to be a valid supported image.
<?xml version="1.0" encoding="utf-8"?> <layer-list xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@color/splash_background" /> <item > <bitmap android:gravity="center|clip_vertical" android:src="@drawable/splash_icon" android:tileMode="disabled" /> </item> </layer-list>
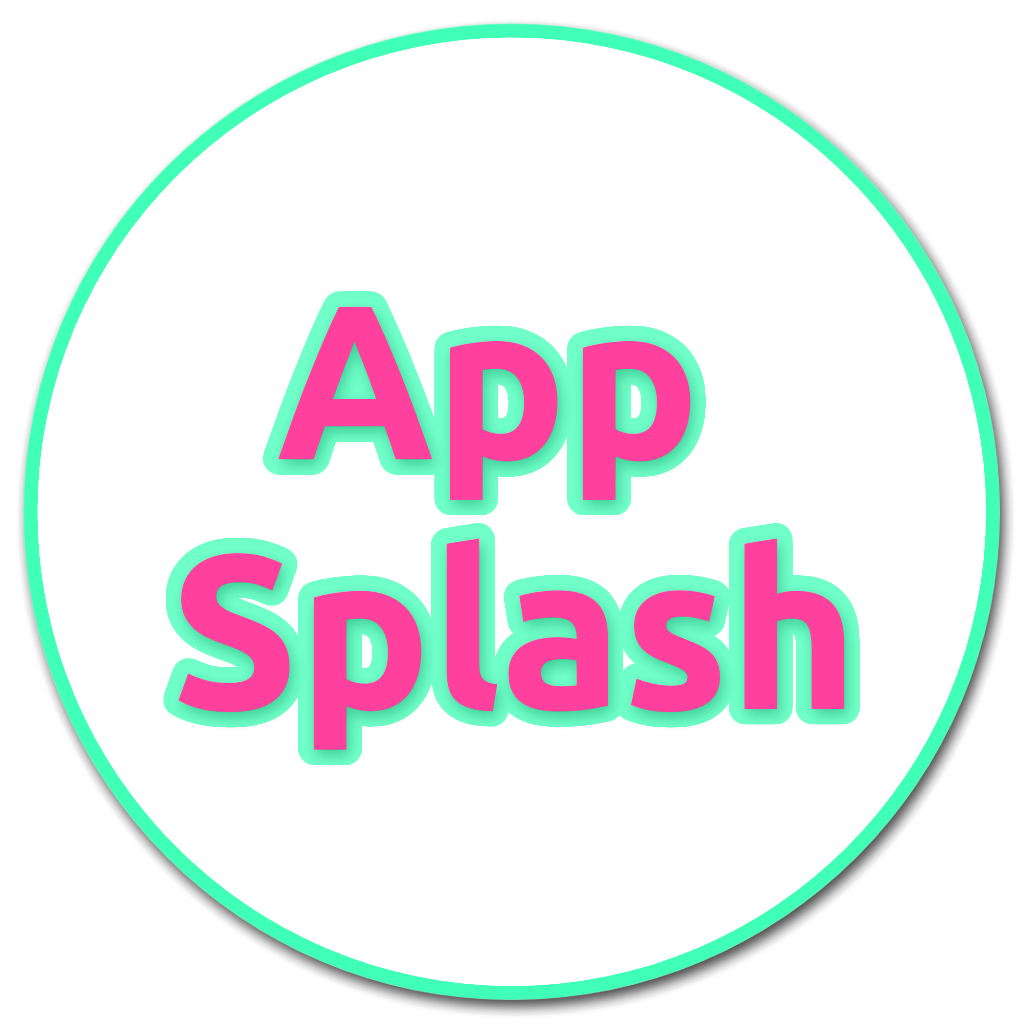
Theme Style
With the drawable created, a splash theme, need to be added to the styles.xml. The drawable is added by adding the item with the attribute name android:windowBackground. Where the id for the drawable is added inside the item object.
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="Splash" parent="Theme.SplashScreen"> <item name="android:windowBackground">@drawable/splash</item> </style> </resources>
Activity
Android does not support any default splash screen. Instead, it has to be done by the developers. By using a dedicated activity that will call the main entry activity for the app. All the while displaying an image as the splash screen during loading.
package org.linuxsenpai.konachan.activity; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.util.Log; /** * Responsible for creating an * activity for which displays the * splash screen of the program while * loading the resources in the background. */ public class SplashActivity extends Activity { static public final String TAG = "Splash"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); /* Create main activity. */ Log.d(TAG, "Creating Activity intent."); Intent intent = new Intent(this, MainActivity.class); startActivity(intent); Log.d(TAG, "Finishing Splash."); /* Close activity. */ finish(); } }
Finally, with everything setup, the activity needs to be added to the AndroidManifest.xml, It is setup as it was commonly setup. However, the most important attribute is the android:theme, which needs to be assigned with the theme style, which will add the splash drawable.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="org.sample.splashscreen"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.SplashScreen"> <activity android:name=".SplashAcitivity" android:theme="@style/Splash"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".MainActivity"> </activity> </application> </manifest>
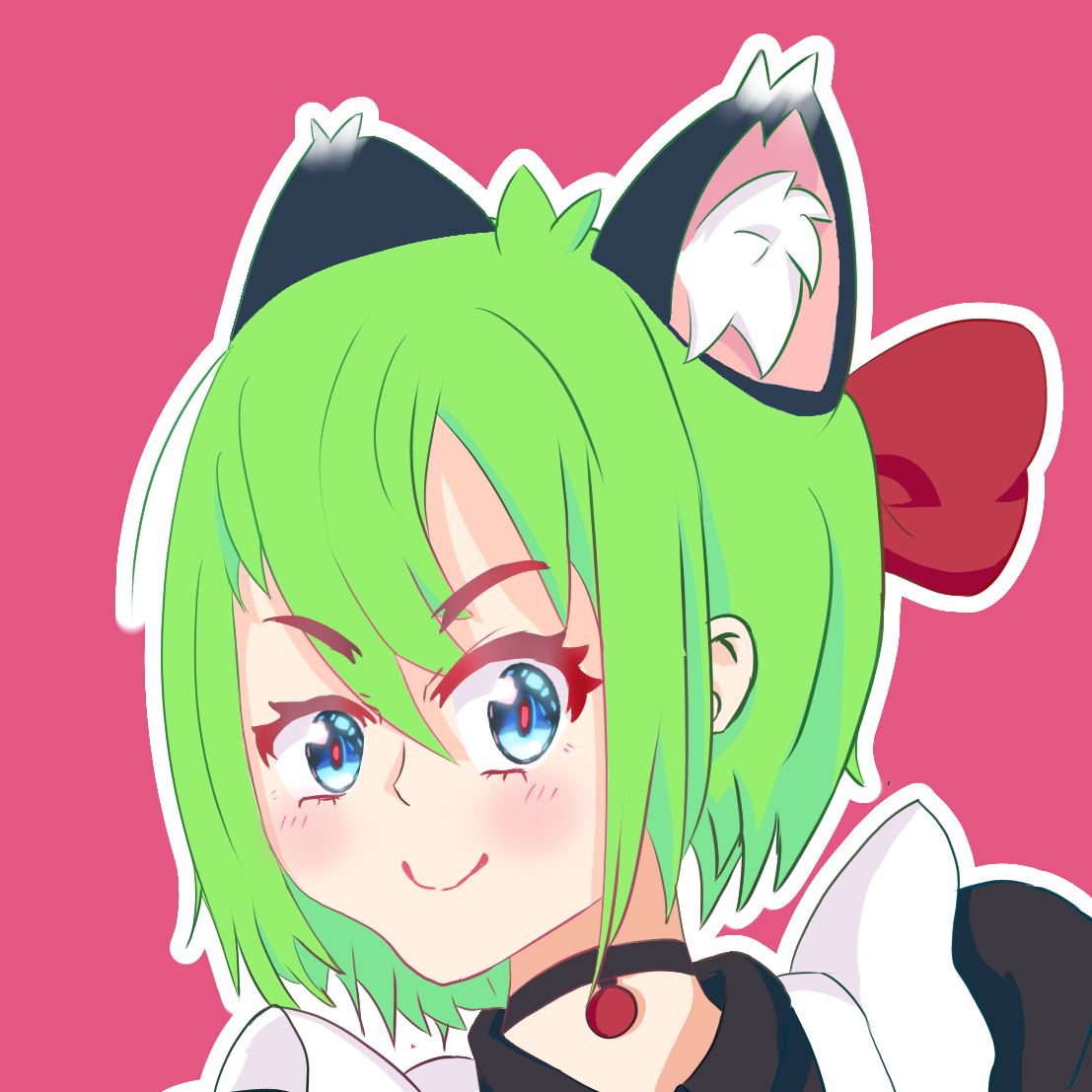
Free/Open software developer, Linux user, Graphic C/C++ software developer, network & hardware enthusiast.