continuous integration and continuous deployment (CI/CD) can be a very crucial part of any software development. Especially if it is used as a product. Let us take a look at how can utilize CI/CD and integrate it as part of our development process, by using GitLab’s CI/CD feature. Let us start scripting.
Basic Understanding
The following is a very simple example, for compiling code, using CMake.
stages: - build build:ubuntu: stage: build tags: - ubuntu script: - mkdir build - cd build - cmake .. - make -j4
Keywords
Here are some common keywords used in GitLab CI/CD scripts.
- stages – Set all the stages that will be part of the CI/CD
- stage – The specific stage, followed by its configuraiton.
- needs – Set which order stages shall be executed. For instance. build stage before test stage
- tags – Set tag associated with the stages, that is related to the gilab-runner, for which runner it shall use for this stage.
- variables – allow to set variables associated with the stage.
- before_script – Set of command that are executable before the script.
- script – executes the set of command associated with the command.
- artifacts – Allow to upload data from the runner to the gitlab, that can be downloaded later.
- image – Allow to set which docker image the stage shall use. For instance, perhaps a image that has all the latex setup, instead of downlading all the package each.
- cache – Allow to setup cache rule between stage, for improving the time it stage takes.
Git Submodules
When working with projects using GIt, many developers choose to use submodules, which is the feature that allows adding a sub git repo, as part of the main git repo. But when using CI/CD we want to make sure they are fetched before running the scripts.
GIT_SUBMODULE_STRATEGY: recursive
build:ubuntu: stage: build tags: - ubuntu variables: GIT_SUBMODULE_STRATEGY: recursive script: - mkdir build - cd build - cmake .. - make -j4
Artifacts
By using artifacts you can perform continuous deployment for your project. It allows to specifiy what files it shall upload and how long they shall remain. Of course, there are many other options for performing the continuous deployment. However, being able to have access to the latest can be very useful.
The Artifacts should be part of the a stage, and it in practice, as one of the last stages, if everything was succesful. It support adding multiple paths as well wildcard. Futhemore, it is possible to explicity set the expiration time.
artifacts:
expire_in: 4 hrs
paths:
- ./*.exe
Caching
A way to improve the runner is by utulizing cache, espeically having many stages. when adding cache as a global, it will beome as the default, unless the stage has specificied its own cache rule.
By adding a set of path, the will automcatially save the content when transition to the next stage.
Example of use case would be for instance, apt packages and build data.
cache: paths: - build/ - apt-cache/ key: apt-cache
Global Common Stages
When creating multiple stages, there might be common steps that they might use. By creating
If for instance, for each of the stags within your CI/CD script, it is possible to create a global default. This will be created outside the scope of a stage. However, if for instance before_script is added to a stage, it will completely override the default global before_script.
stages: - build - test before_script: - export DEBIAN_FRONTEND=noninteractive - apt-get update -yq - apt-get install --yes --no-install-recommends - apt-get install -y clang-10 g++-10 cmake make build:ubuntu: stage: build tags: - ubuntu script: - mkdir build - cd build - cmake .. - make test:ubuntu: stage: build tags: - ubuntu script: - mkdir build - cd build - cmake .. - make
Multiple Stages
Example of using multiple stages
stages: - build - test - doc - publish build:ubuntu: stage: build tags: - ubuntu script: - mkdir build - cd build - cmake .. - make test:ubuntu: stage: build needs: build tags: - ubuntu script: - mkdir build - cd build - cmake .. -DBUILD_TEST - make - ctest doc:ubuntu: stage: build tags: - ubuntu script: - ./generateDoc.sh test:ubuntu: stage: build needs: build tags: - ubuntu script: - mkdir build - cd build - cmake .. -DBUILD_TEST - make - ctest
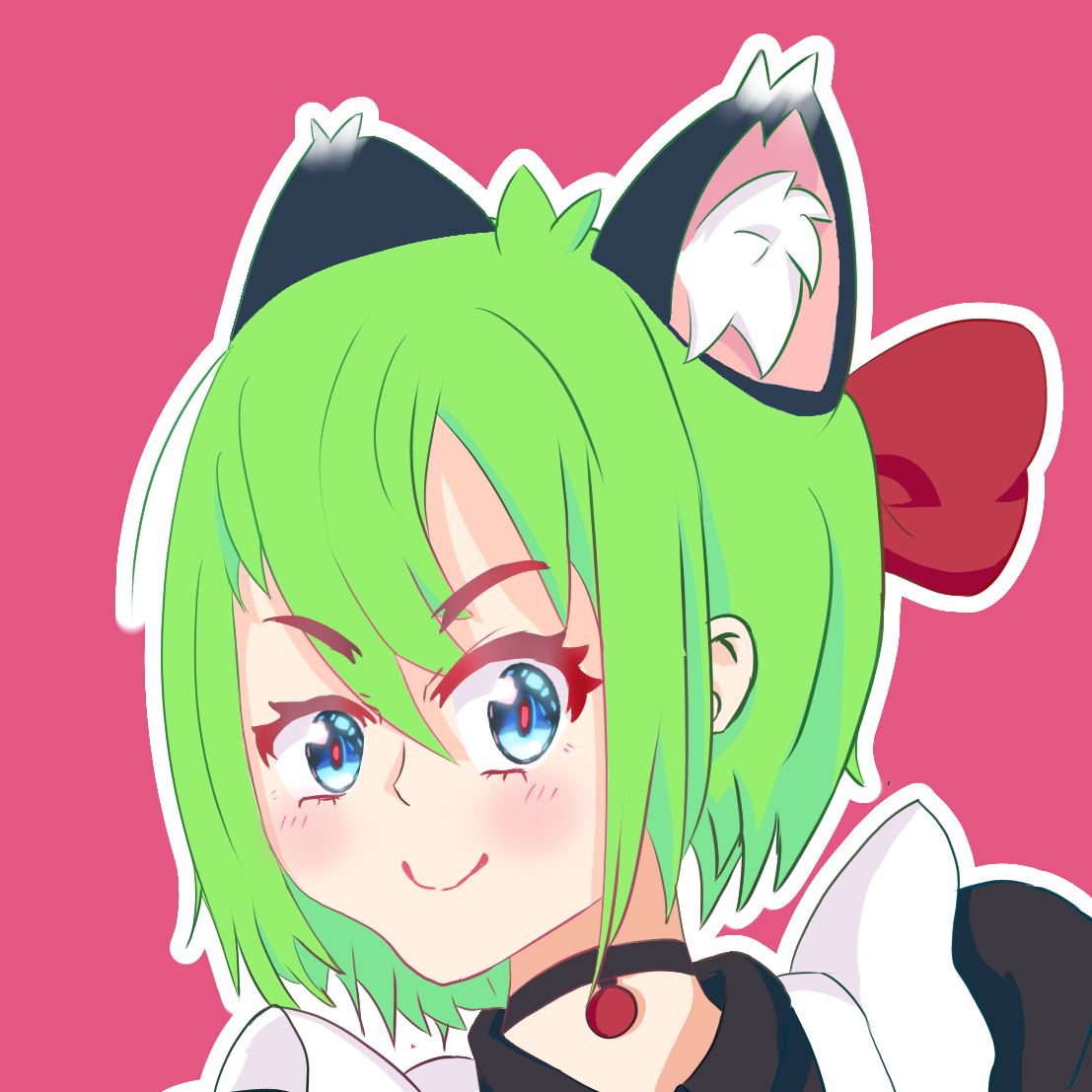
Free/Open software developer, Linux user, Graphic C/C++ software developer, network & hardware enthusiast.