In Android development, Activities can be simplified as being viewed as a container object for which everything visible on the current application is contained. However, for more detail view the android reference documentation. Here, I will only attempt to show how to start a android activity and pass data with code examples. The source code can be found at GitHub.
Activities can be used for instance creating a home view and settings view, where each of them is independent of each other. Another example could be using an activity for the splash screen while loading the main activity.
Simple Start Activity
Let us start with the most simple startActivity example. Where we intend to start an activity from other activity class within our own application. The Intent class name is based on the fact we intend to start an activity. Thus, it will not guarantee it will start the activity. It will default fail, see the next section to configure the application to allow for it to start the activity
@Override protected void onCreate(Bundle savedInstanceState) { Intent intent = new Intent(this, MyActivity.class); startActivity(intent); }
Android Application Configuration File
Important, before the Android application will accept the activity in the previous section. It needs to be included within the AndroidManifest.xml file. Each activity in the application needs to be specified within the application tag in order to be allowed to start.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="org.sample.startactivity"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.StartActivity"> <activity android:name=".MyActivity"> </activity> <activity android:name=".MainActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Passing Data Between Activities
Since activities are can be viewed as an independent objects. Thus, do not really communicate directly with each other. However, activities can pass data to actives and get result status. But that is the extent of their communication. Thus we need to know how to pass information. Fortunately, this can be done easily by using the intent and bundle, which is optional since the intent has the same method that the bundle class.
The arguments are passed similar to a directory data class. Each key-value is associated with a value in order for the other activity that is receiving the data can find and process the data accordingly.
import android.content.Intent; import android.os.Bundle; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); Intent intent = new Intent(this, MyActivity.class); /* */ Bundle bundle = new Bundle(); /* Optional, same method can be used with the intent. */ bundle.putInt("myInt", 42); bundle.putString("myString", "Hello World"); /* */ intent.putExtras(bundle); startActivity(intent); } }
Retrieve Data Between Activities
Now, after having created a bundle of data and started the activity Let us see how we can extract the information from the other activity. All we need is to use the getIntent method and simply extract the bundle from it, using the same key word. The savedInstacedState is of a bundle type as well. However, it contains a different kinds of data.
import android.content.Intent; import android.os.Bundle; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; public class MyActivity extends AppCompatActivity { @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); Bundle bundle = getIntent().getExtras(); int intValue = bundle.getInt("MyInt"); String stringValue = bundle.getString("myString"); } }
Start External Activities
Finally, let us see how our application can ask the Android system to start another application activity based on the data we pass send via the intent. We can see instantly it uses a string constant via the Intent class instead of the class type object. Next, it uses the putExtra directly via the intent object instead of a bundle. It will pass the data to the bundle directly. Finally, by using the string constant query, all other activities that listen to the argument can get the correct information.
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Intent intent = new Intent(Intent.ACTION_SEARCH); intent.putExtra(SearchManager.QUERY, "cat"); startActivity(intent); }
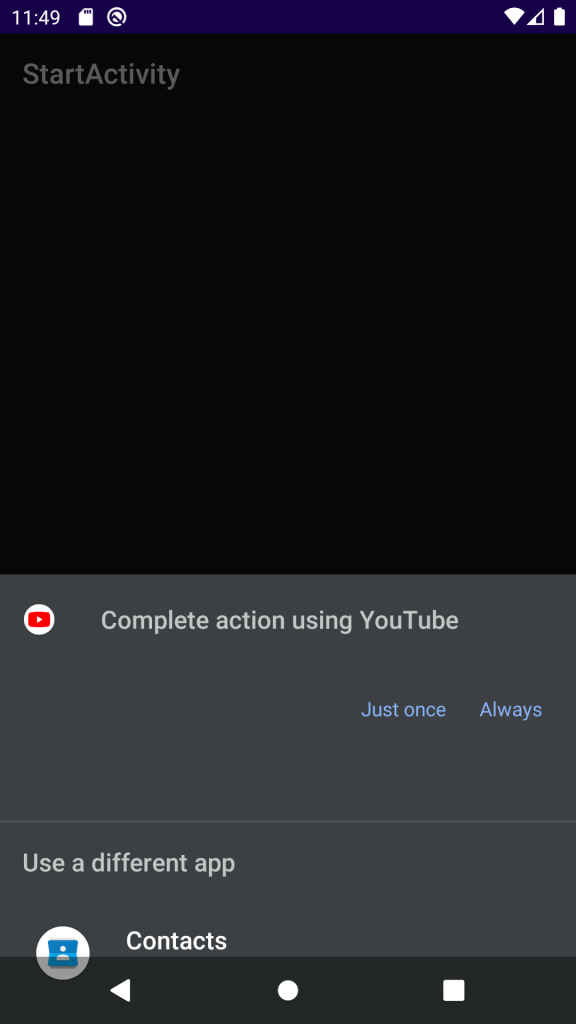
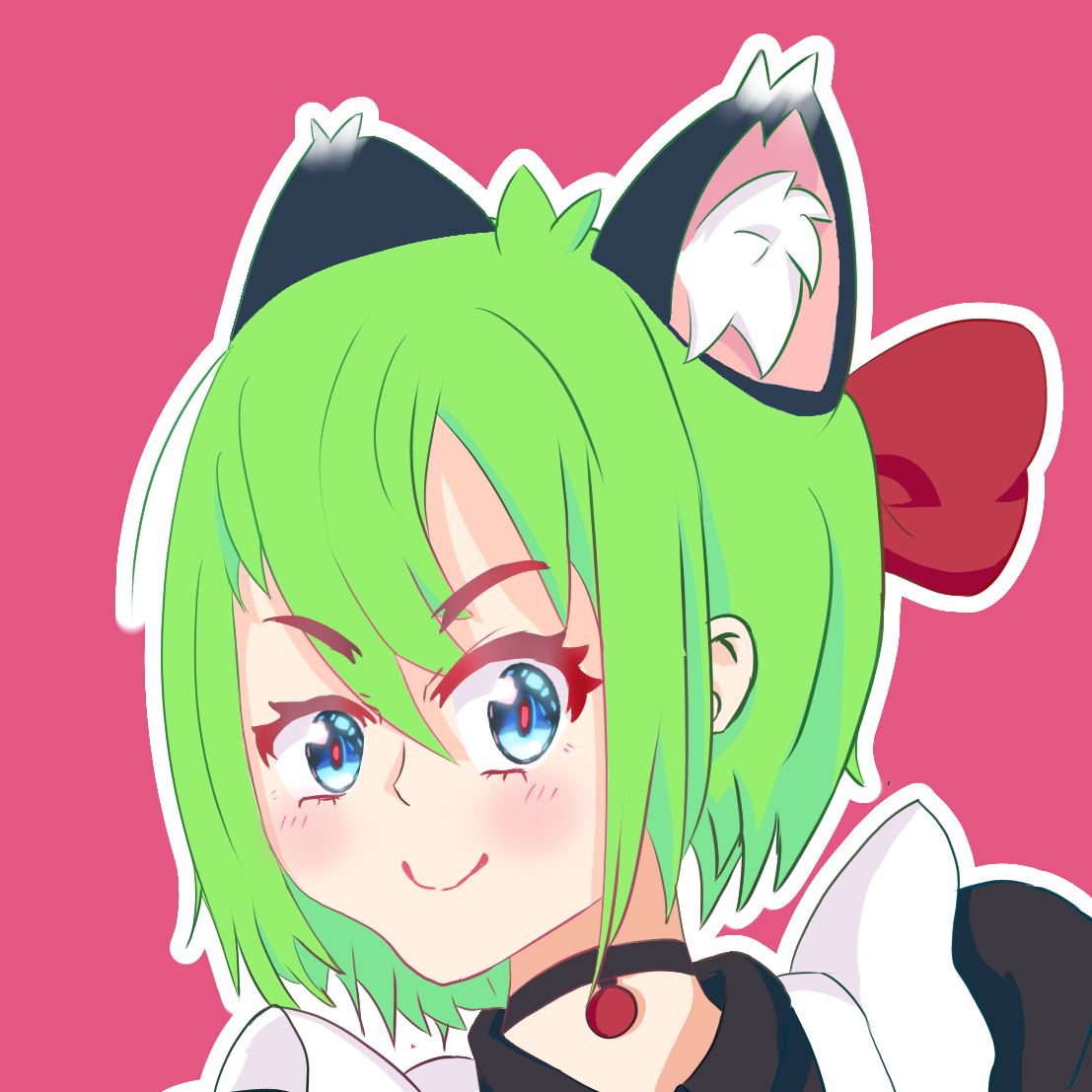
Free/Open software developer, Linux user, Graphic C/C++ software developer, network & hardware enthusiast.